{ "cells": [ { "cell_type": "markdown", "source": [ "# Input Output Data Handling\n", "\n", "First you need to understand the difference between \"State Input\" and \"Action Input\". The \"State Input\" is just the \"State Output\" from previous state. And the Action is the underlying computation, such as Lambda, ECS, Glue. The \"Action Input\" is the raw JSON input for the computation.\n", "\n", "AWS Step Function Provides the following method to manipulate the Input/Output data:\n", "\n", "- ``InputPath``: convert \"State Input\" to \"Action Input\", select single JSON node.\n", "- ``Parameters``: convert \"State Input\" to \"Action Input\", use payload template language to construct the \"Action Input\".\n", "- ``ResultSelector``: convert \"Action Output\" to \"State Output\", use payload template language to construct the \"State Output\".\n", "- ``ResultPath``: convert \"Action Output\" to \"State Output\", select single JSON node.\n", "\n", "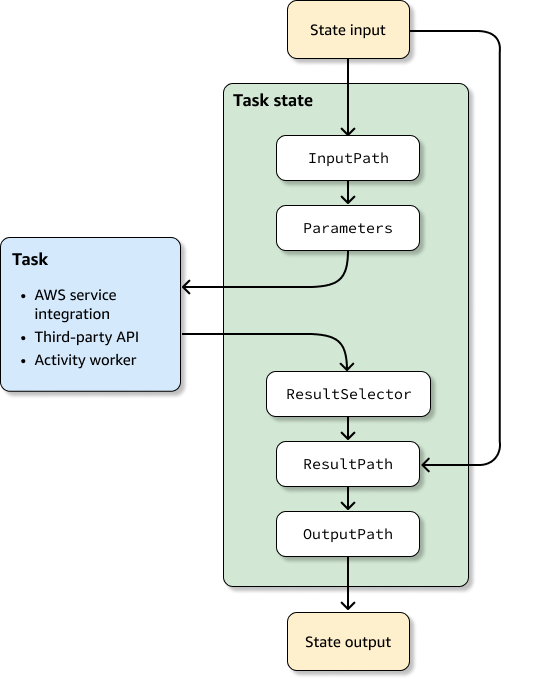\n", "\n", "In this example, we create a simple EShop purchase use case. First we would like to convert the state input from the API, extract the payment information, and \"Verify Payment\". If payment is verified, we would like to forward the \"order_id\" information and send to \"Process Order\". If payment is NOT verified, we fail the workflow." ], "metadata": { "collapsed": false, "pycharm": { "name": "#%% md\n" } } }, { "cell_type": "code", "execution_count": 5, "outputs": [], "source": [ "import aws_stepfunction as sfn\n", "from rich import print" ], "metadata": { "collapsed": false, "pycharm": { "name": "#%%\n" } } }, { "cell_type": "markdown", "source": [ "" ], "metadata": { "collapsed": false, "pycharm": { "name": "#%% md\n" } } }, { "cell_type": "code", "execution_count": 6, "outputs": [], "source": [ "workflow = sfn.Workflow()\n", "\n", "verify_payment = sfn.Task(\n", " id=\"Verify Payment\",\n", " resource=\"arn:...\",\n", " parameters={\n", " \"credit_card_number\": \"$.payment.card_number\",\n", " \"order_id\": \"$.order.order_id\",\n", " },\n", " result_selector={\n", " \"verified\": \"$.verify_result.result\",\n", " \"order_id\": \"$.order_id\",\n", " },\n", ")\n", "\n", "process_order = sfn.Task(\n", " id=\"Process Order\",\n", " resource=\"arn:...\",\n", " parameters={\n", " \"order_id\": \"$.order_id\",\n", " },\n", ")" ], "metadata": { "collapsed": false, "pycharm": { "name": "#%%\n" } } }, { "cell_type": "code", "execution_count": 7, "outputs": [ { "data": { "text/plain": "\u001B[1m{\u001B[0m\n \u001B[32m'StartAt'\u001B[0m: \u001B[32m'Verify Payment'\u001B[0m,\n \u001B[32m'States'\u001B[0m: \u001B[1m{\u001B[0m\n \u001B[32m'Verify Payment'\u001B[0m: \u001B[1m{\u001B[0m\n \u001B[32m'Type'\u001B[0m: \u001B[32m'Task'\u001B[0m,\n \u001B[32m'Resource'\u001B[0m: \u001B[32m'arn:...'\u001B[0m,\n \u001B[32m'Next'\u001B[0m: \u001B[32m'Choice-by-Verify Payment'\u001B[0m,\n \u001B[32m'Parameters'\u001B[0m: \u001B[1m{\u001B[0m\u001B[32m'credit_card_number'\u001B[0m: \u001B[32m'$.payment.card_number'\u001B[0m, \u001B[32m'order_id'\u001B[0m: \u001B[32m'$.order.order_id'\u001B[0m\u001B[1m}\u001B[0m,\n \u001B[32m'ResultSelector'\u001B[0m: \u001B[1m{\u001B[0m\u001B[32m'verified'\u001B[0m: \u001B[32m'$.verify_result.result'\u001B[0m, \u001B[32m'order_id'\u001B[0m: \u001B[32m'$.order_id'\u001B[0m\u001B[1m}\u001B[0m\n \u001B[1m}\u001B[0m,\n \u001B[32m'Choice-by-Verify Payment'\u001B[0m: \u001B[1m{\u001B[0m\n \u001B[32m'Type'\u001B[0m: \u001B[32m'Choice'\u001B[0m,\n \u001B[32m'Choices'\u001B[0m: \u001B[1m[\u001B[0m\u001B[1m{\u001B[0m\u001B[32m'Variable'\u001B[0m: \u001B[32m'$.verified'\u001B[0m, \u001B[32m'BooleanEquals'\u001B[0m: \u001B[3;92mTrue\u001B[0m, \u001B[32m'Next'\u001B[0m: \u001B[32m'Process Order'\u001B[0m\u001B[1m}\u001B[0m\u001B[1m]\u001B[0m,\n \u001B[32m'Default'\u001B[0m: \u001B[32m'Fail-0c3c4cb'\u001B[0m\n \u001B[1m}\u001B[0m,\n \u001B[32m'Process Order'\u001B[0m: \u001B[1m{\u001B[0m\n \u001B[32m'Type'\u001B[0m: \u001B[32m'Task'\u001B[0m,\n \u001B[32m'Resource'\u001B[0m: \u001B[32m'arn:...'\u001B[0m,\n \u001B[32m'End'\u001B[0m: \u001B[3;92mTrue\u001B[0m,\n \u001B[32m'Parameters'\u001B[0m: \u001B[1m{\u001B[0m\u001B[32m'order_id'\u001B[0m: \u001B[32m'$.order_id'\u001B[0m\u001B[1m}\u001B[0m\n \u001B[1m}\u001B[0m,\n \u001B[32m'Fail-0c3c4cb'\u001B[0m: \u001B[1m{\u001B[0m\u001B[32m'Type'\u001B[0m: \u001B[32m'Fail'\u001B[0m\u001B[1m}\u001B[0m\n \u001B[1m}\u001B[0m\n\u001B[1m}\u001B[0m\n", "text/html": "
{\n 'StartAt': 'Verify Payment',\n 'States': {\n 'Verify Payment': {\n 'Type': 'Task',\n 'Resource': 'arn:...',\n 'Next': 'Choice-by-Verify Payment',\n 'Parameters': {'credit_card_number': '$.payment.card_number', 'order_id': '$.order.order_id'},\n 'ResultSelector': {'verified': '$.verify_result.result', 'order_id': '$.order_id'}\n },\n 'Choice-by-Verify Payment': {\n 'Type': 'Choice',\n 'Choices': [{'Variable': '$.verified', 'BooleanEquals': True, 'Next': 'Process Order'}],\n 'Default': 'Fail-0c3c4cb'\n },\n 'Process Order': {\n 'Type': 'Task',\n 'Resource': 'arn:...',\n 'End': True,\n 'Parameters': {'order_id': '$.order_id'}\n },\n 'Fail-0c3c4cb': {'Type': 'Fail'}\n }\n}\n\n" }, "metadata": {}, "output_type": "display_data" } ], "source": [ "(\n", " workflow.start_from(verify_payment)\n", " .choice(\n", " [\n", " sfn.Var(\"$.verified\").boolean_equals(True)\n", " .next_then(process_order)\n", " ],\n", " default=sfn.Fail(),\n", " )\n", ")\n", "\n", "workflow.continue_from(process_order).end()\n", "\n", "print(workflow.serialize())" ], "metadata": { "collapsed": false, "pycharm": { "name": "#%%\n" } } }, { "cell_type": "code", "execution_count": null, "outputs": [], "source": [], "metadata": { "collapsed": false, "pycharm": { "name": "#%%\n" } } } ], "metadata": { "kernelspec": { "display_name": "Python 3", "language": "python", "name": "python3" }, "language_info": { "codemirror_mode": { "name": "ipython", "version": 2 }, "file_extension": ".py", "mimetype": "text/x-python", "name": "python", "nbconvert_exporter": "python", "pygments_lexer": "ipython2", "version": "2.7.6" } }, "nbformat": 4, "nbformat_minor": 0 }